mvpa2.clfs.base.AttributeMap¶
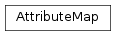
-
class
mvpa2.clfs.base.
AttributeMap
(map=None, mapnumeric=False, collisions_resolution=None)¶ Map to translate literal values to numeric ones (and back).
A translation map is derived automatically from the argument of the first call to to_numeric(). The default mapping is to map unique value (in sorted order) to increasing integer values starting from zero.
In case the default mapping is undesired a custom map can be specified to the constructor call.
Regardless of how the mapping has been specified or derived, it remains constant (i.e. it is not influenced by subsequent calls to meth:
to_numeric
or meth:to_literal
. However, the translation map can be removed with meth:clear
.Both conversion methods take sequence-like input and return arrays.
Examples
Default mapping procedure using an automatically derived translation map:
>>> am = AttributeMap() >>> am.to_numeric(['eins', 'zwei', 'drei']) array([1, 2, 0])
>>> print am.to_literal([1, 2, 0]) ['eins', 'zwei', 'drei']
Custom mapping:
>>> am = AttributeMap(map={'eins': 11, 'zwei': 22, 'drei': 33}) >>> am.to_numeric(['eins', 'zwei', 'drei']) array([11, 22, 33])
Methods
clear
()Remove previously established mappings. items
()Dict-like generator yielding literal/numerical pairs. iteritems
()Dict-like generator yielding literal/numerical pairs. keys
()Returns the literal names of the attribute map. to_literal
(attr[, recurse])Map numerical value back to literal ones. to_numeric
(attr)Map literal attribute values to numerical ones. values
()Returns the numerical values of the attribute map. Parameters: map : dict
Custom dict with literal keys mapping to numerical values.
mapnumeric : bool
In some cases it is necessary to map numeric labels too, for instance when target labels should be from a specific set, e.g. (-1, +1).
collisions_resolution : None or {‘tuple’, ‘lucky’}
How to resolve collisions on to_literal if multiple entries map to the same value when custom map was provided. If None – exception would get raise, if ‘tuple’ – collided entries are grouped into a tuple, if ‘lucky’ – some last encountered literal wins (i.e. arbitrary resolution). This parameter is in effect only when calling
to_literal()
.Please see the class documentation for more information.
Methods
clear
()Remove previously established mappings. items
()Dict-like generator yielding literal/numerical pairs. iteritems
()Dict-like generator yielding literal/numerical pairs. keys
()Returns the literal names of the attribute map. to_literal
(attr[, recurse])Map numerical value back to literal ones. to_numeric
(attr)Map literal attribute values to numerical ones. values
()Returns the numerical values of the attribute map. -
clear
()¶ Remove previously established mappings.
-
items
()¶ Dict-like generator yielding literal/numerical pairs.
-
iteritems
()¶ Dict-like generator yielding literal/numerical pairs.
-
keys
()¶ Returns the literal names of the attribute map.
-
to_literal
(attr, recurse=False)¶ Map numerical value back to literal ones.
Parameters: attr : sequence
Numerical values to be mapped.
recurse : bool
Either to recursively change items within the sequence if those are iterable as well
Please see the class documentation for more information.
-
to_numeric
(attr)¶ Map literal attribute values to numerical ones.
Arguments with numerical data type will be returned as is.
Parameters: attr : sequence
Literal values to be mapped.
Please see the class documentation for more information.
-
values
()¶ Returns the numerical values of the attribute map.
-