mvpa2.misc.surfing.volume_mask_dict.VolumeMaskDictionary¶
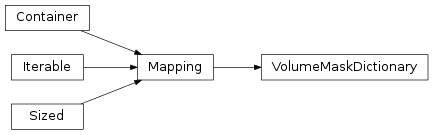
-
class
mvpa2.misc.surfing.volume_mask_dict.
VolumeMaskDictionary
(vg, source, meta=None, src2nbr=None, src2aux=None)¶ Collection of 3D volume masks, indexed by integers or strings.
Voxels in a mask are represented sparsely, that is by linear indices in the [0, n-1] range, where n is the number of voxels in the volume.
A typical use case is storing voxel selection results, which can subsequently be used for running searchlights. An alternative use case is storing a set of regions-of-interest masks.
Because this class extends Mapping, it can be indexed as any other mapping (like dicts). Currently masks cannot be removed, however, and adding masks is performed through the add(...) function rather than item assignment.
In some functions the terminology ‘source’ and ‘target’ is used. A source refers to the label associated with masks (and can be used as a key in the mapping), while a target is an element from the value associated in this mapping (i.e. typically a target is a linear voxel index).
Besides storing voxel indices, each mask can also have additional‘auxiliary’ information associated, such as distance from the center or its relative position in grey matter.
Attributes
meta
Return meta information such as number of node indices source
Geometric information of (the centers of) each mask. volgeom
Volume geometry information. Methods
add
(src, nbrs[, aux])Add a volume mask aux_get
(\*args, \*\*kwargs)DEPRECATED: use .get_aux instead aux_keys
()Names of auxiliary labels get
(src)Return the linear voxel indices of a mask get_aux
(src, label)Auxiliary information of a mask get_dataset_feature_mask
(ds[, keys])For a dataset return a mask of features that were selected get_mask
([keys])Return a mask for voxels that are included in one or more masks get_minimal_dataset
(ds[, keys])For a dataset return only portion with features which were selected get_nifti_image_mask
([keys])Return a NIfTI image with the voxels included in any mask get_targets
()Return list of voxels that are in one or more masks get_tuple_list
(src, \*labels)Return a list of tuples with mask indices and/or aux information. get_tuple_list_dict
(\*args, \*\*kwargs)DEPRECATED: use .get_tuple_list instead get_voxel_indices
([keys])Returns voxel indices at least once selected is_same_layout
(other)Check whether another instance has the same spatial layout items
(() -> list of D’s (key, value) pairs, ...)iteritems
(() -> an iterator over the (key, ...)iterkeys
(() -> an iterator over the keys of D)itervalues
(...)keys
(() -> list of D’s keys)merge
(other)Add masks from another instance source2nearest_target
(source)Find the voxel nearest to a mask center target2nearest_source
(target[, ...])Find the voxel nearest to a mask center target2sources
(nbr)Find the indices of masks that map to a linear voxel index values
(() -> list of D’s values)xyz_source
([ss])Computes the x,y,z coordinates of one or more mask centers xyz_target
([ts])Compute the x,y,z coordinates of one or more voxels Initialize a VolumeMaskDictionary
Parameters: vg: volgeom.VolGeom or fmri_dataset-like or str
data structure that contains volume geometry information.
source: Surface.surf or numpy.ndarray or None
structure that contains the geometric information of (the centers of) each mask. In the case of surface-searchlights this should be a surface used as the center for searchlights.
meta: dict or None
Optional meta data stored with this instance (such as searchlight radius and volumetric information). A use case is storing an instance and loading it later, and then checking whether the meta information is correct when it used to run a searchlight analysis.
src2nbr: dict or None
In a typical use case it contains a mapping from node center indices to lists of voxel indices.
src2aux: dict or None
In a typical use case it can contain auxiliary information such as distance of each voxel to each center.
Attributes
meta
Return meta information such as number of node indices source
Geometric information of (the centers of) each mask. volgeom
Volume geometry information. Methods
add
(src, nbrs[, aux])Add a volume mask aux_get
(\*args, \*\*kwargs)DEPRECATED: use .get_aux instead aux_keys
()Names of auxiliary labels get
(src)Return the linear voxel indices of a mask get_aux
(src, label)Auxiliary information of a mask get_dataset_feature_mask
(ds[, keys])For a dataset return a mask of features that were selected get_mask
([keys])Return a mask for voxels that are included in one or more masks get_minimal_dataset
(ds[, keys])For a dataset return only portion with features which were selected get_nifti_image_mask
([keys])Return a NIfTI image with the voxels included in any mask get_targets
()Return list of voxels that are in one or more masks get_tuple_list
(src, \*labels)Return a list of tuples with mask indices and/or aux information. get_tuple_list_dict
(\*args, \*\*kwargs)DEPRECATED: use .get_tuple_list instead get_voxel_indices
([keys])Returns voxel indices at least once selected is_same_layout
(other)Check whether another instance has the same spatial layout items
(() -> list of D’s (key, value) pairs, ...)iteritems
(() -> an iterator over the (key, ...)iterkeys
(() -> an iterator over the keys of D)itervalues
(...)keys
(() -> list of D’s keys)merge
(other)Add masks from another instance source2nearest_target
(source)Find the voxel nearest to a mask center target2nearest_source
(target[, ...])Find the voxel nearest to a mask center target2sources
(nbr)Find the indices of masks that map to a linear voxel index values
(() -> list of D’s values)xyz_source
([ss])Computes the x,y,z coordinates of one or more mask centers xyz_target
([ts])Compute the x,y,z coordinates of one or more voxels -
add
(src, nbrs, aux=None)¶ Add a volume mask
Parameters: src: int or str
index or name of volume mask. src should not be already present in this dictionary
nbrs: list of int
linear voxel indices of the voxels in the mask
aux: dict or None
auxiliary properties associated with (the voxels in) the volume mask. If the current dictionary instance alraedy has stored auxiliary properties for other masks, then the set of keys in the current mask should be the same as for other masks. In addition, the length of each value in aux should be either the number of elements in nbrs or one.
-
aux_get
(*args, **kwargs)¶ DEPRECATED: use .get_aux instead
-
aux_keys
()¶ Names of auxiliary labels
Returns: keys: list of str
Names of auxiliary labels that are supported by get_aux
-
get
(src)¶ Return the linear voxel indices of a mask
Parameters: src: int
index of mask
Returns: idxs: list of int
linear voxel indices indexed by src
-
get_aux
(src, label)¶ Auxiliary information of a mask
Parameters: src: int
index of mask
label: str
label of auxiliary information
Returns: vals: list
auxiliary information labelled label for mask src
-
get_dataset_feature_mask
(ds, keys=None)¶ For a dataset return a mask of features that were selected at least once
Parameters: ds: Dataset
A dataset with field .fa.voxel_indices
keys: list or None
Indices of center ids for which the associated masks must be used. If None, all keys are used.
Returns: mask: np.ndarray (boolean)
binary mask with ds.nfeatures values, with True for features that were selected at least once once since the initalization of this class. That is, for a voxel (feature) with feature index i it holds that mask[I] is True iff there is at least one key k so that i in self.get(k).
Notes
When using surface-based searchlights, a use case of this function is to get the voxels that were associated with the searchlights in a subset of all nodes on a cortical surface.
-
get_mask
(keys=None)¶ Return a mask for voxels that are included in one or more masks
Parameters: keys: list or None
Indices of center ids for which the associated masks must be used. If None, all keys are used.
Returns: msk: np.ndarray
Three-dimensional array with True for voxels that are included in one or more masks, and False elsewhere
Notes
When using surface-based searchlights, a use case of this function is to get the voxels that were associated with the searchlights in a subset of all nodes on a cortical surface.
-
get_minimal_dataset
(ds, keys=None)¶ For a dataset return only portion with features which were selected
Parameters: ds: Dataset
A dataset with field .fa.voxel_indices
keys: list or None
Indices of center ids for which the associated masks must be used. If None, all keys are used.
Returns: Dataset
A dataset containing features that were selected at least once
Notes
The rationale of this function is that voxel selection can be run first (without using a mask for a dataset), then the dataset can be reduced to contain only voxels that were selected by voxel selection
-
get_nifti_image_mask
(keys=None)¶ Return a NIfTI image with the voxels included in any mask
Parameters: keys: list or None
Indices of center ids for which the associated masks must be used. If None, all keys are used.
Returns: msk: nibabel.Nifti1Image
Nifti image where voxels have the value 1 for voxels that are included in one or more masks, and 0 elsewhere
Notes
When using surface-based searchlights, a use case of this function is to get the voxels that were associated with the searchlights in a subset of all nodes on a cortical surface.
-
get_targets
()¶ Return list of voxels that are in one or more masks
Returns: idxs: list of int
Linear indices of voxels in one or more masks
-
get_tuple_list
(src, *labels)¶ Return a list of tuples with mask indices and/or aux information.
Parameters: src: int or str
index of mask
*labels: str or None
List of labels to return. None refers to the voxel indices of the mask.
Returns: tuples: list of tuple
N tuples each with len(labels) values, where N is the number of voxels in the mask indexed by src
-
get_tuple_list_dict
(*args, **kwargs)¶ DEPRECATED: use .get_tuple_list instead
- Return a dictionary of mapping that maps each mask index
- to tuples with mask indices and/or auxiliary information.
Parameters: *labels: str or None
List of labels to return. None refers to the voxel indices of the mask.
- tuple_dict: dict
a mapping so that get_tuple_list(s, labels)==get_tuple_list_dict(labels)[s]
-
get_voxel_indices
(keys=None)¶ Returns voxel indices at least once selected
Parameters: keys: list or None
Indices of center ids for which the associated masks must be used. If None, all keys are used.
Returns: voxel_indices: list of tuple
List of triples with sub-voxel indices that were selected at least once since the initalization of this class. That is, a triple (i,j,k) referring to a voxel V is an element of voxel_indices iff there is at least one key k so that self.get(k) contains the linear index of voxel V.
Notes
When using surface-based searchlights, a use case of this function is to get the voxels that were associated with the searchlights in a subset of all nodes on a cortical surface.
-
is_same_layout
(other)¶ Check whether another instance has the same spatial layout
Parameters: other: VolumeMaskDictionary
the instance that is compared to the current instance
Returns: same: boolean
True iff the other instance has the same volume geometry and source as the current instance
-
merge
(other)¶ Add masks from another instance
Parameters: other: VolumeMaskDictionary
The instance from which masks are added to the current one. The keys in the current and other instance should be disjoint, and auxiliary properties (if present) should have the same labels.
-
meta
¶ Return meta information such as number of node indices
Returns: meta: dict or False
dictionary with meta information, or False if no such information is present. The latter case is to be compatible with old-style instances loaded with h5load
-
source
¶ Geometric information of (the centers of) each mask.
In the case of surface-searchlights this should be a surface used as the center for searchlights.
-
source2nearest_target
(source)¶ Find the voxel nearest to a mask center
Parameters: src: int
mask index
Returns: target: int
linear index of the voxel that is contained in the mask associated with src and nearest (Euclidean distance) to src.
-
target2nearest_source
(target, fallback_euclidean_distance=False)¶ Find the voxel nearest to a mask center
Parameters: target: int
linear index of a voxel
fallback_euclidean_distance: bool (default: False)
Whether to use a euclidean distance metric if target is not in any of the masks in this instance
Returns: src: int
key index for the mask that contains target and is nearest to target. If target is not contained in any mask, then None is returned if fallback_euclidean_distance is False, and the index of the source nearest to target using a Euclidean distance metric is returned if fallback_euclidean_distance is True
-
target2sources
(nbr)¶ Find the indices of masks that map to a linear voxel index
Parameters: nbr: int
Linear voxel index
Returns: srcs: list of int
Indices i for which get(i) contains nbr
-
volgeom
¶ Volume geometry information.
-
xyz_source
(ss=None)¶ Computes the x,y,z coordinates of one or more mask centers
Parameters: ss: list of int or None
list of mask center indices for which coordinates should be computed. If ss is None, then coordinates for all masks that are mapped are computed. If is required that when the current instance was initialized, the source-argument was either a surf.Surface or a numpy.ndarray.
-
xyz_target
(ts=None)¶ Compute the x,y,z coordinates of one or more voxels
Parameters: ts: list of int or None
list of voxels for which coordinates should be computed. If ts is None, then coordinates for all voxels that are mapped are computed
Returns: xyz: numpy.ndarray
Array with size len(ts) x 3 with x,y,z coordinates
-